Data Alerts
On this page:
About Data Alerts
Data Alerts is a large UI component that shows a list of configured Data Alerts and contains a modal that enables the creation of new Data Alerts.
Tip: This widget works best if placed within a full page.
Custom Data Alerts are User-Level Data Alerts, which means they can be created, edited and deleted by the End User, from the widget itself.
To create a new Data Alert or edit an existing one, a modal that contains a simple setup workflow is also included within this widget. Within the modal, the user is prompted to set up a customized Data Alert by following this series of steps:
- Choose Alert Type
- Set Up Conditions
- Configure Timing
- Customize Appearance
To set up an alert, the user must query the parameters in either Data Messenger or a Dashboard tile to create their Alert.
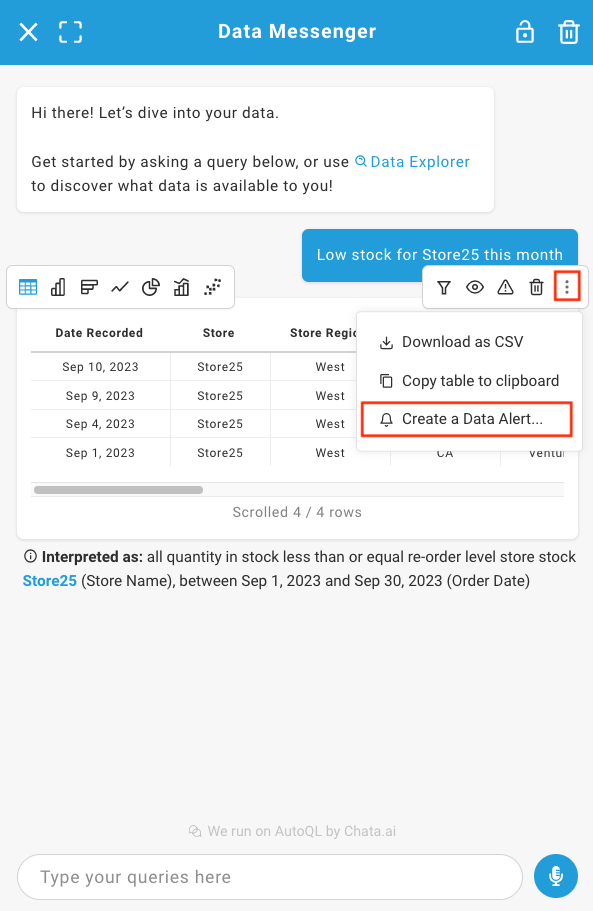
The first step is to choose the alert type: Live Alert, which notifies you when data meets specific conditions, or Scheduled Alert, which sends updates at set intervals. Select the appropriate option and click Next to proceed.
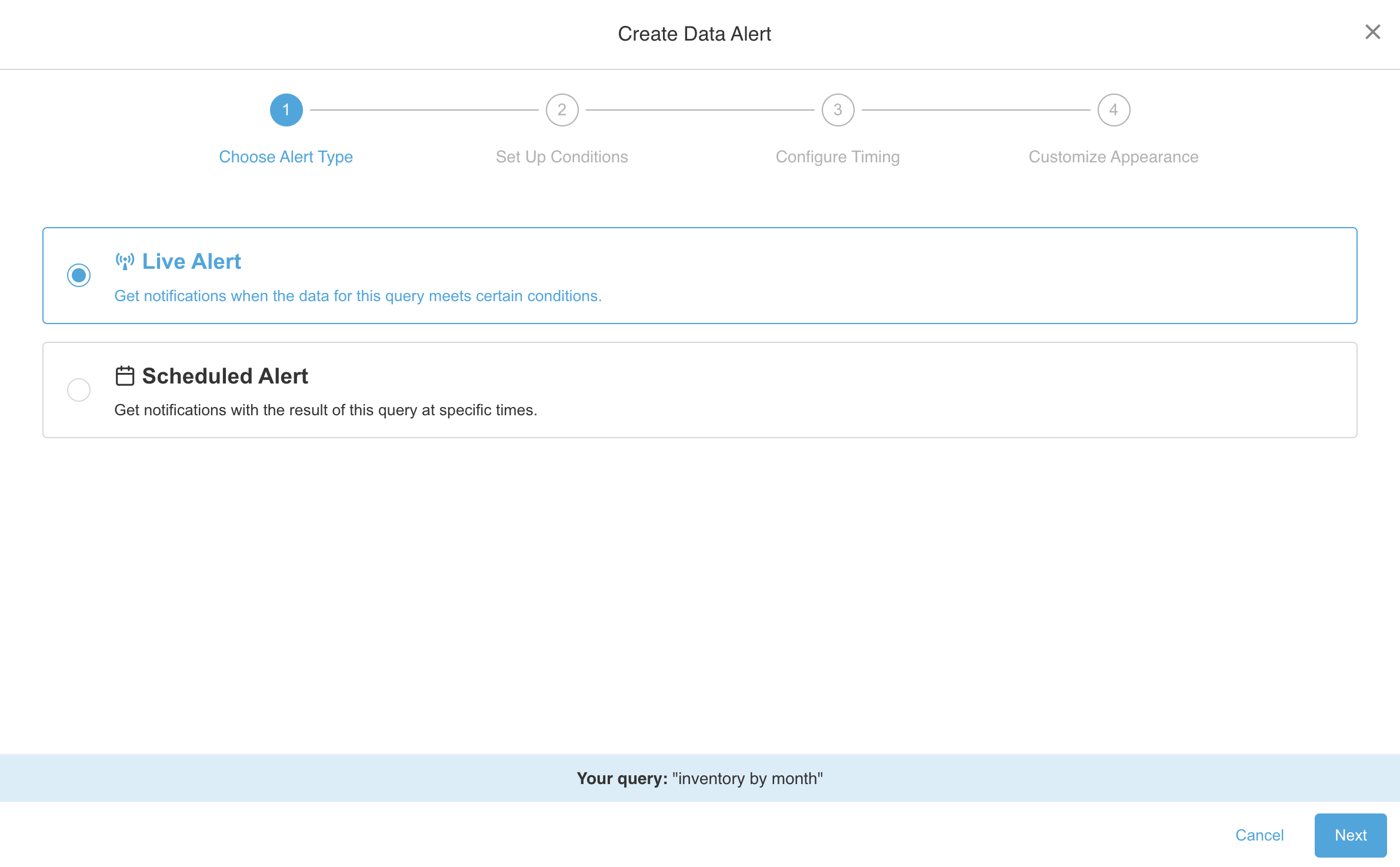
The second step is to set up conditions. You can choose to receive a notification when the query receives new rows of data or contains data that meets specific conditions. The query being monitored is "Inventory by month", and the user can set a condition based on the Quantity in Stock column. The second image shows that the condition can compare the selected column against a specific number, the result of another query, or another column from the same query (Coming feature). Once the condition is set, the user can proceed by clicking Next, return to the previous step with Back, or cancel the setup using Cancel.
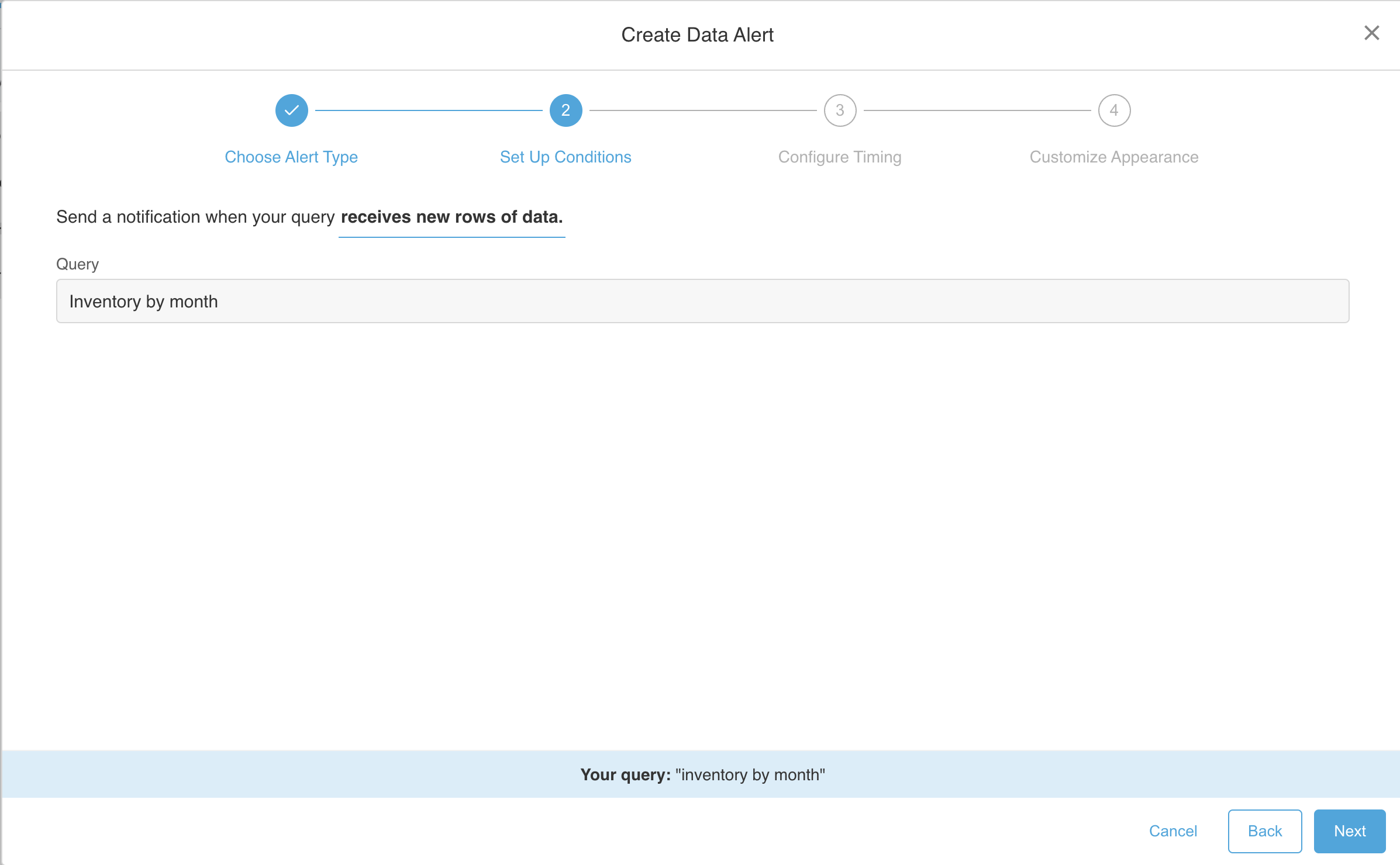
- When the query receives new rows of data.
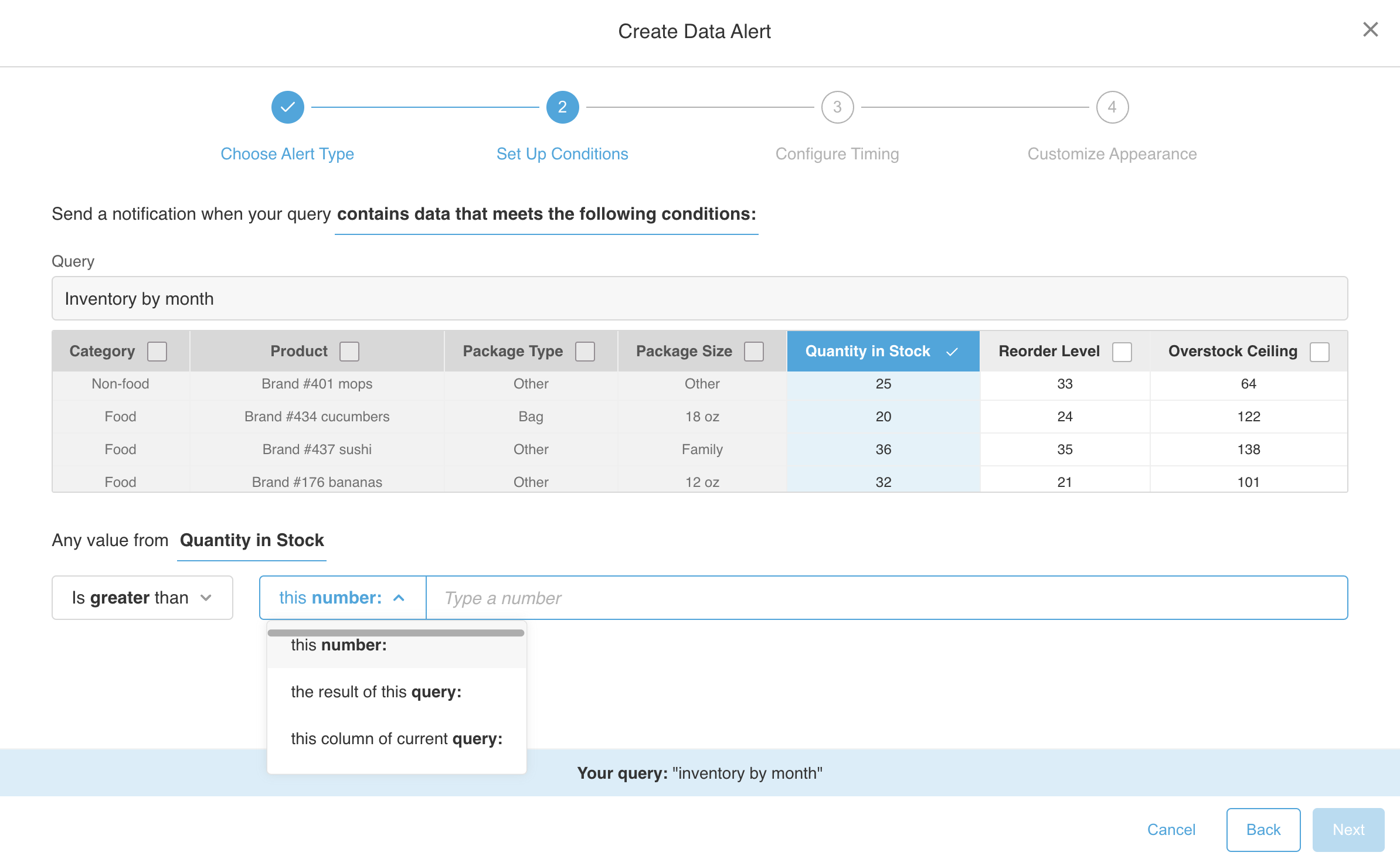
- When the query contains data that meets specific conditions.
Next, the user will be prompted to schedule the frequency at which they wish to be notified if the conditions of their new Data Alert are met. They can opt to be notified right away (when the event happens), or be notified every time that event happens. They can also set up the Alert so they are only notified at specific intervals: Daily, Weekly, or Monthly.
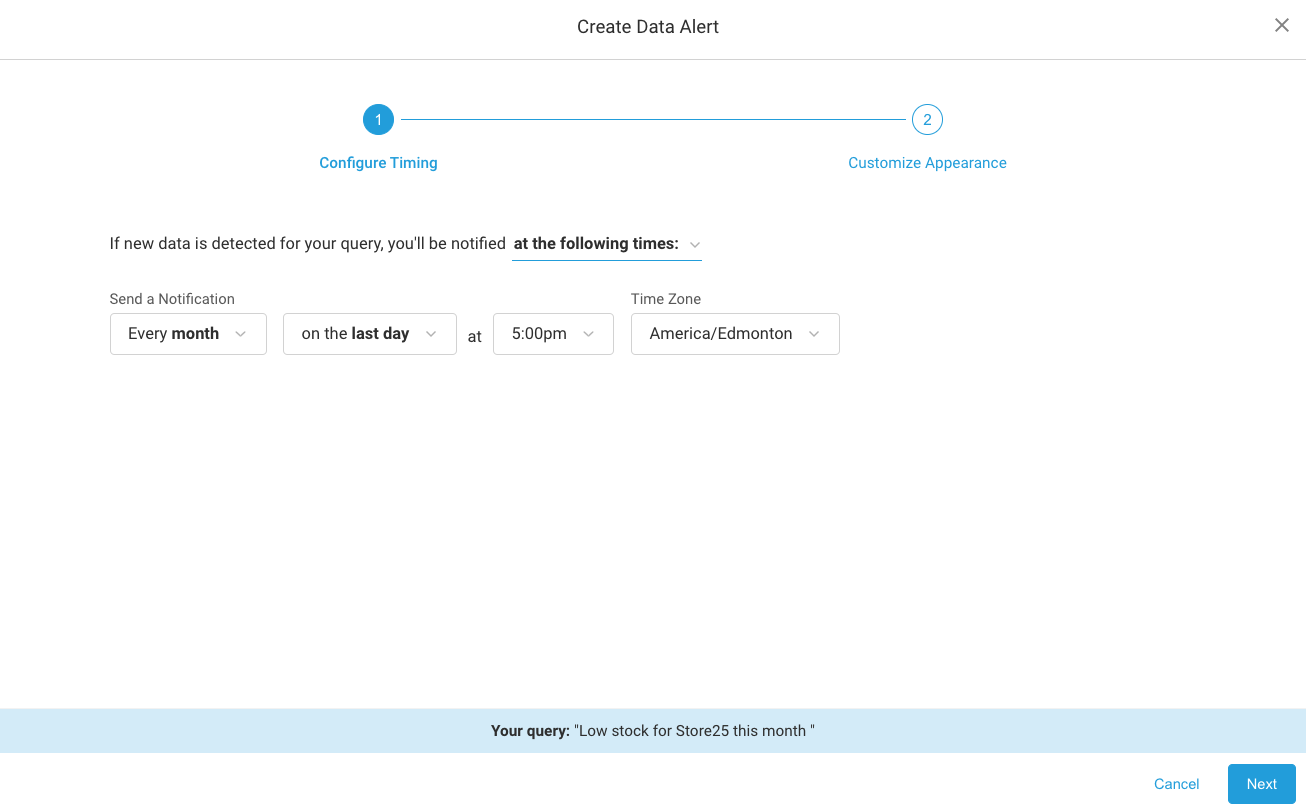
Next, the user sets up their Alert Custom Preferences. Here, the user can use natural language to specify the data response they wish to receive when their Alert is triggered. They can also add a customized message to provide helpful context when the notification is received.
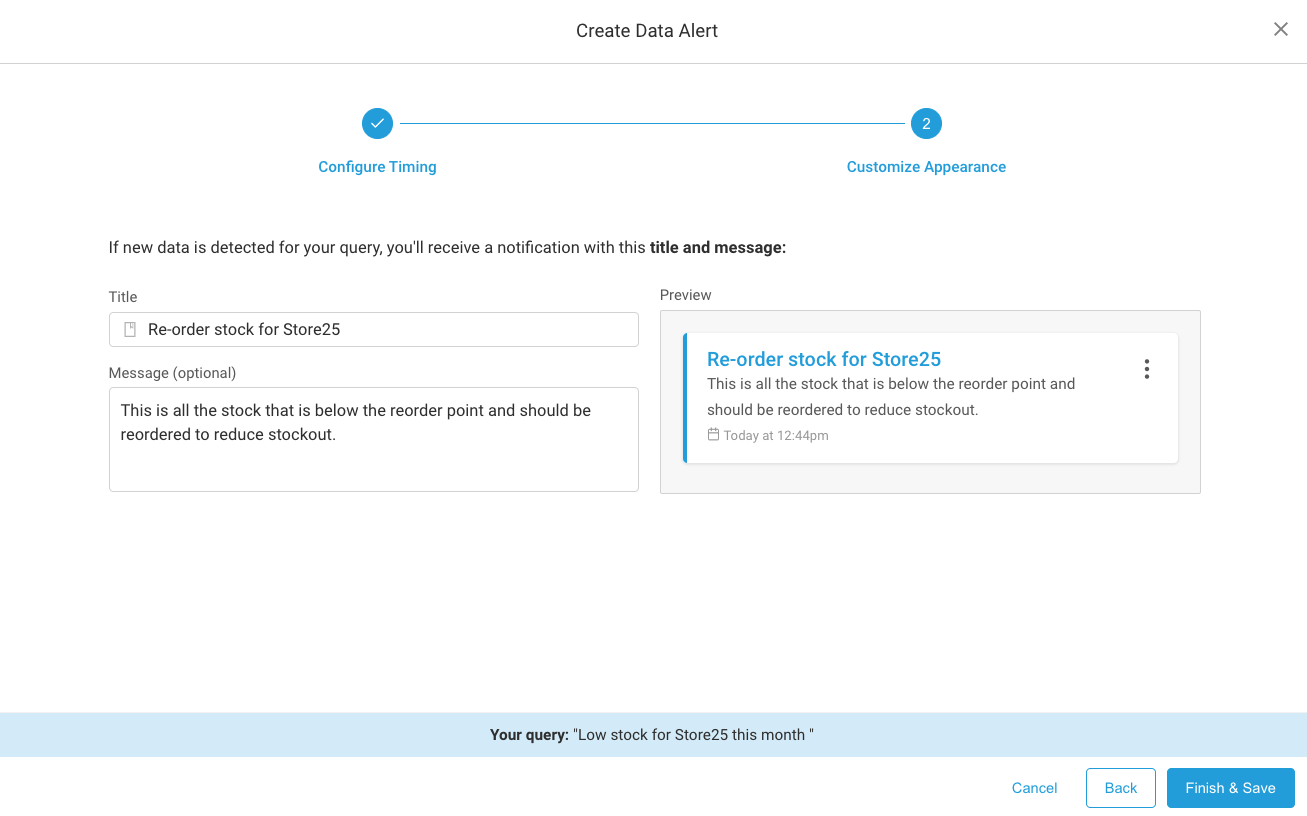
Setting Up Data Alerts
The following sections in this document contain detailed information about how to customize the properties of this widget.
To get started, simply import the DataAlerts component and pass in the required authentication prop:
import React from 'react'
import { DataAlerts } from 'react-autoql';
import 'react-autoql/dist/autoql.esm.css'
export default class App extends React.Component {
render = () => {
return (
<DataAlerts
authentication={{
apiKey="your-api-key"
domain="https://yourdomain.com"
token="your-jwt-token"
}}
/>
)
}
}
Props
Prop Name | Data Type | Default Value |
---|---|---|
authentication (required) | Object | {} |
Object | {} | |
onSuccessAlert | Function | (message)=>{} |
onErrorCallback | Function | (error) => {} |
onDMLinkClick | Function | ()=>{} |
onDashboardLinkClick | Function | ()=>{} |
onErrorCallback(error)
: A callback for when an error occurs in the component.
onSuccessAlert(message)
: A callback for when a successful alert occurs in the component.
onDMLinkClick()
: A callback for when a Data Messenger link is clicked in the component.
onDashboardLinkClick()
: A callback for when a Dashboard link is clicked in the component.
authentication Prop
Key | Value Type | Description |
---|---|---|
token | String | Your valid JWT encrypted with your user ID and customer ID. For more information on how to create this token, please see: Create JSON Web Tokens (JWT) |
apiKey | String | Your API key. For more information on how to obtain this, please see: Manage API Keys |
domain | String | The base URL for your API. |
Examples
import React from 'react'
import { DataAlerts } from 'react-autoql';
import 'react-autoql/dist/autoql.esm.css'
export default class App extends React.Component {
render = () => {
return (
<DataAlerts
authentication={{
apiKey="your-api-key"
domain="https://yourdomain.com"
token="your-jwt-token"
}}
onErrorCallback={(error) => {
if (error.message) alert(error.message)
}}
onSuccessAlert={(message)=>{
if (message) alert(message)
}}
/>
)
}
}
Updated 4 months ago