Data Messenger
Deliver the power of AutoQL to your users through Data Messenger, a state-of-the-art conversational interface you can easily build into your existing application.
On this page:
About Data Messenger
Data Messenger is a conversational user interface (CUI). It is a flexible front-end widget that can be implemented and accessed within an existing application or software interface. Data Messenger enables users to interact conversationally with their data by entering natural language queries to receive information from their database (or more simply, allows users to ask questions in their own words to receive data-related responses).
Throughout this document, the words "Data Messenger" and "widget" are used interchangeably. All widget components are open source, so Data Messenger is highly customizable in terms of both function and design.
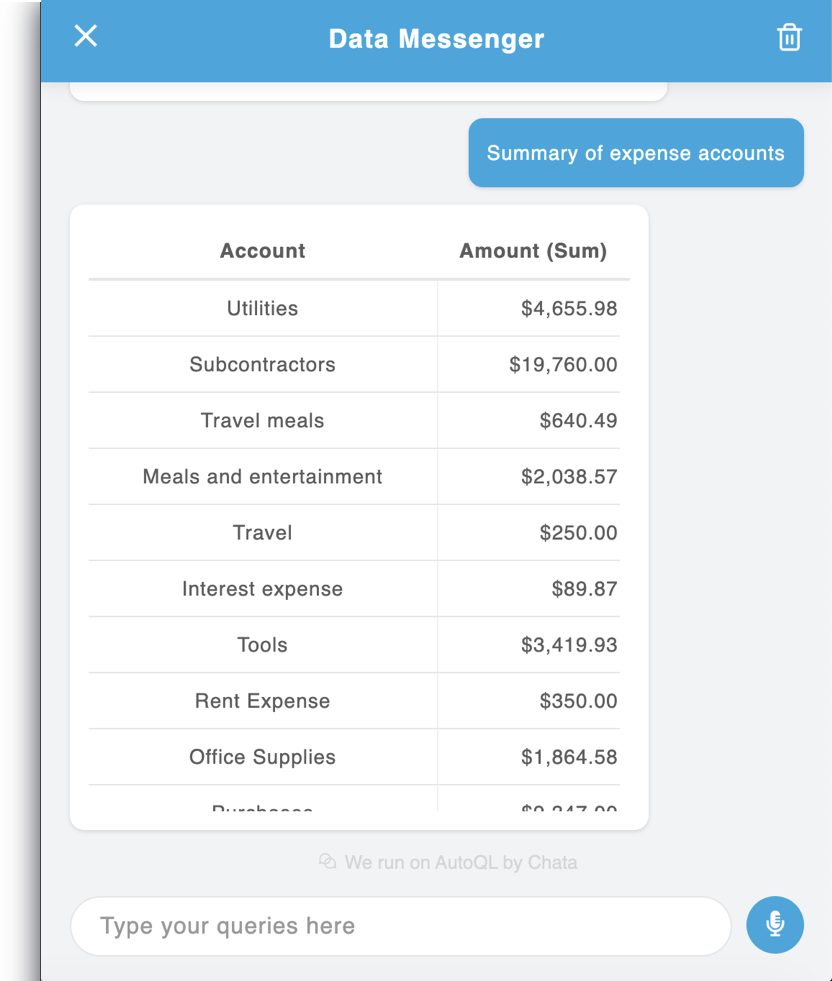
Setting Up Data Messenger
The following sections in this document contain detailed information about how to customize the properties of the widget.
import { DataMessenger } from 'react-autoql'
import 'react-autoql/dist/autoql.esm.css'
const MyComponent = () => {
return (
<DataMessenger
authentication={{
apiKey: 'your-api-key',
domain: 'https://yourdomain.com',
token: 'yourToken',
}}
/>
)
}
export default MyComponent
import React, { Component } from 'react'
import { DataMessenger } from 'react-autoql';
import 'react-autoql/dist/autoql.esm.css'
export default class MyComponent extends Component {
render = () => {
return (
<DataMessenger
authentication={{
apiKey: "your-api-key",
domain: "https://yourdomain.com",
token: "yourToken"
}}
/>
)
}
}
Props
Prop Name | Data Type | Default Value |
---|---|---|
authentication | Object | {} |
placement | String: 'left' || 'right' || 'top' || 'bottom' | 'right' |
enableExploreQueriesTab | Boolean | true |
enableNotificationsTab | Boolean | false |
landingPage | String: 'data-messenger' || 'explore-queries' | 'data-messenger' |
width | String || Number | 500 |
height | String || Number | 350 |
resizable | Boolean | true |
title | String | 'Data Messenger' |
userDisplayName | String | 'there' |
introMessage | String | 'Hi ${userDisplayName}! I'm here to help you access, search and analyze your data.' |
inputValue | String | undefined |
enableQueryQuickStartTopics | Boolean | true |
inputPlaceholder | String | 'Type your queries here' |
showMask | Boolean | true |
maskClosable | Boolean | true |
onMaskClick | Function | onHandleClick |
shiftScreen | Boolean | false |
onVisibleChange | Function | () => {} |
showHandle | Boolean | true |
handleStyles | Object | {} |
maxMessages | Number | undefined |
clearOnClose | Boolean | false |
enableVoiceRecord | Boolean | true |
enableDynamicCharting | Boolean | true |
autoChartAggregations | Boolean | true |
autocompleteStyles | Object | {} |
autoQLConfig | Object | {} |
dataFormatting | Object | {} |
themeConfig | Object | {} |
placement
: Determines the edge of the screen where Data Messenger is placed.
enableExploreQueriesTab
: Explore Queries is a separate view within Data Messenger that provides a custom, searchable catalogue of natural language queries to get your users started, fast. To use it, the user simply types in a keyword or topic to search for related queries. Queries related to the input from the user are returned and the user can click on any query they wish to run. The selected query will then be run automatically within the default Data Messenger view.
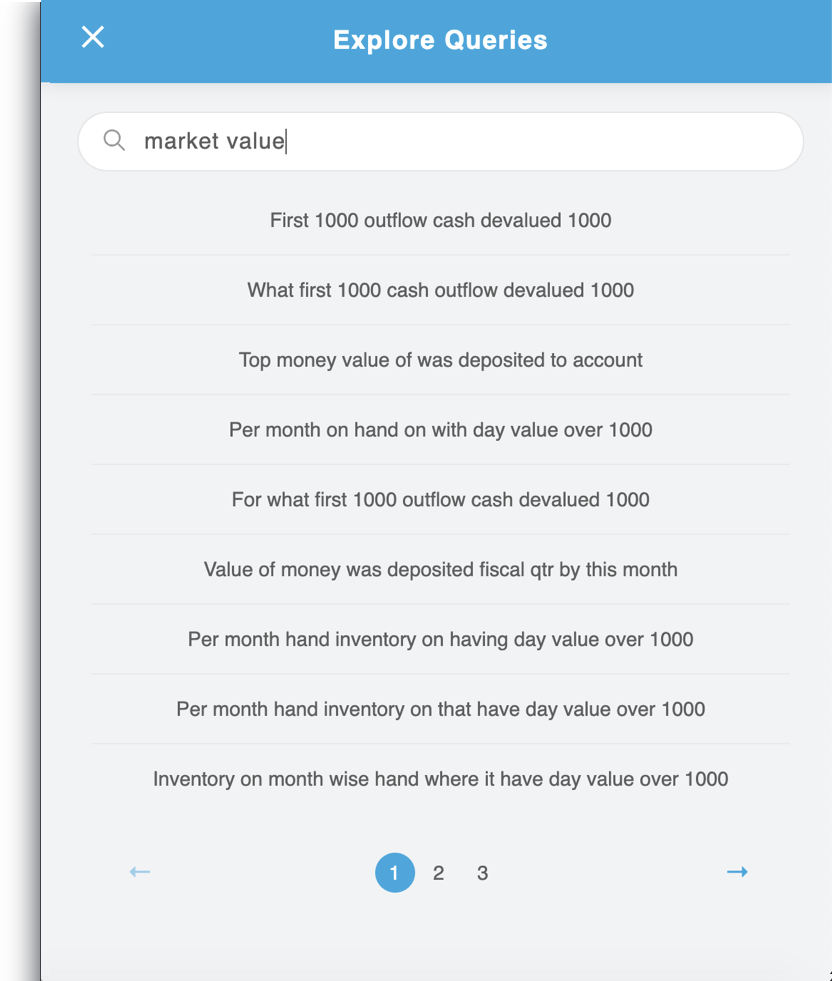
enableNotificationsTab
: Notifications tab is a separate view within Data Messenger that provides a feed for Data Alert Notifications in tandem with your data alerts integration. For more details see Notification Feed.
landingPage
: Choose which tab to begin on when Data Messenger is opened for the first time.
width
: Set the widget width in pixels. If the value is larger than the screen width, it will default to the screen width. This value will only be applied for placements "left" and "right". The value will be ignored for "top" and "bottom" placements. If the resizable prop is "true", this value will serve as the initial width only.
height
: Set the height of the widget in pixels. If the value is larger than the screen height, it will default to the screen height. This value will only be applied for "top" and "bottom" placements. This value will be ignored for "left" and "right" placements. If the resizable prop is "true", this value will serve as the initial height only.
resizable
: If this prop is "true", Data Messenger will be dynamically resizable. Users can easily change the size of the widget by clicking and dragging from the edge.
title
: Text that appears in the header of the default Data Messenger view. You must provide an empty string if you do not want text to appear here, otherwise the default text (Data Messenger) will apply.
userDisplayName
: Name used in the intro message (ex. "Hi Nikki!"). You can customize this value using names from your own database.
introMessage
: Customize the default intro message using your own brand voice and custom copy. The userDisplayName prop will be ignored if this is provided.
inputValue
: Controlled input text. This is useful for when you want to populate the input field by clicking something outside of the messenger.
enableQueryQuickStartTopics
: When true (default), Data Messenger fetches a list of predefined query topics and a concise list of natural language queries related to each topic. The user can simply click on a query to get started. If this prop is "false", DataMessenger won't display the QueryQuickStartTopics.
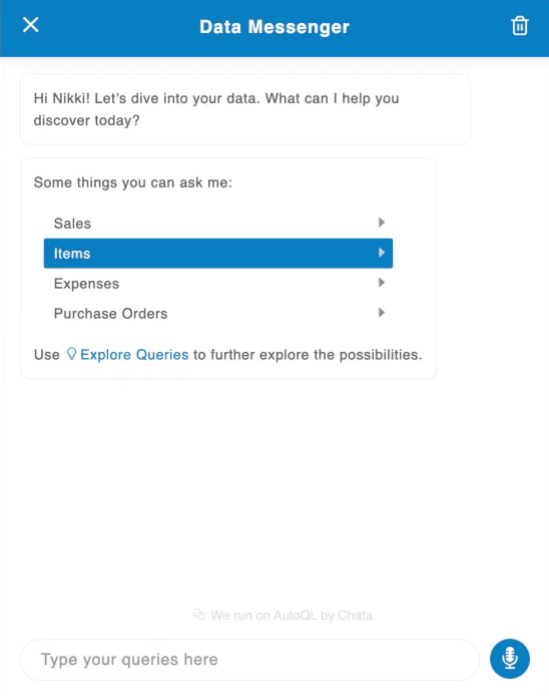
inputPlaceholder
: Customize the placeholder for the Query Input (natural language query search bar).
showMask
: Whether to show the mask (mask presents as a grayed out overlay when Data Messenger is open).
maskClosable
: If this value is set to true, the onHandleClick function will be called when the mask is clicked. If showMask is false, this prop will be ignored.
onMaskClick
: If showMask is true, this is the callback for when the mask is clicked.
shiftScreen
: Whether to shift the whole screen over when the widget opens/closes.
onVisibleChange
: Callback after the widget closes or opens.
showHandle
: Determines whether to show the handle on the edge of the interface. If you do not wish to show the handle, you can use your own custom button and control the widget with the isVisible prop.
handleStyles
: Specify custom CSS styles for the handle. Must pass in a valid JSX CSS style object (ex. { backgroundColor: '#000000' }).
maxMessages
: Maximum number of messages that can be displayed in the Data Messenger interface at one time. A message is any input or any output in the interface. This means a query entered by a user constitutes one message, and the response returned in the Data Messenger interface constitutes another message. If a new message is added and you have reached the maximum, the oldest message will be erased. Note: Any number smaller than 2 will be ignored.
clearOnClose
: Determines whether to clear all messages when the widget is closed. Note: The default intro message will appear when you reopen the widget after closing it.
enableVoiceRecord
: Enables the voice-to-text button. Note: The voice-to-text feature uses Web Speech API, so this feature is only available for Chrome users.
enableDynamicCharting
: Enables the ability to dynamically change the categories for the x and y axes in a chart by clicking on the axis label. If enableDynamicCharting is true and there are other category options available for that axis, an arrow will appear beside the axis label.
autoChartAggregations
: If autoChartAggregations is true, the default display type for aggregation queries will be a chart instead of a table.
autocompleteStyles
: Object with JSX CSS to style the auto-complete popup (ex. { borderRadius: '4px' }).
authentication Prop
Key | Value Type | Description |
---|---|---|
token | String | Your valid JWT encrypted with your user ID and customer ID. For more information on how to create this token, please visit the "Security" section of our docs. |
apiKey | String | Your API key. For more information on how to obtain this, please visit the "Security" section of our docs. |
domain | String | The base URL for your API. For more information on how to obtain this, please visit the "Security" section of our docs. |
autoQLConfig Prop
Key | Value Type | Default |
---|---|---|
enableAutocomplete | Boolean | true |
enableQueryValidation | Boolean | true |
enableQuerySuggestions | Boolean | true |
enableDrilldowns | Boolean | true |
enableColumnVisibilityManager | Boolean | true |
enableQueryInterpretation | Boolean | false |
defaultShowInterpretation | Boolean | false |
enableFilterLocking | Boolean | false |
enableNotifications | Boolean | false |
enableCSVDownload | Boolean | false |
debug | Boolean | false |
enableAutocomplete
: Automatically populates similar query suggestions as users enter a query, so they get results more quickly and easily. If enabled, suggested queries will begin to appear above the Query Input bar as the user types.
enableQueryValidation
: Catches and verifies references to unique data, so users always receive the data they need. If enabled, the natural language query entered by a user will first go through the validate endpoint. If the query requires validation (ex. the input contains reference to a unique data label), suggestions for that label will be returned in a subsequent message, allowing the user to verify their input before executing their query.
For example: If you query, 'How much money does Nikki owe me?', validation may detect that there is no 'Nikki' label, but there are labels called 'Nicki', and 'Nik' in your database. The message will then let you select the appropriate label and run the corresponding query.
If this value is false, the query will bypass the Validate a Query endpoint and be sent straight to the query endpoint.
enableQuerySuggestions
: Enables option for user to clarify meaning in cases where their original query lacked context or could be interpreted in multiple diverse ways. If enabled, in cases where the query input was ambiguous, a list of suggested queries will be returned for the user to choose from, leading to more efficient and accurate responses. If this is false, a general error message will appear in its place.
enableDrilldowns
: When a table or chart element is clicked by a user, a new query will run automatically, allowing the user to "drilldown" into the data to obtain a detailed breakdown of the figure returned by entry. If this is false, nothing will happen when a table or chart element is clicked.
enableColumnVisibilityManager
: Column Visibility Manager allows the user to control the visibility of individual columns when query results are returned in a table. Users can access the Column Visibility Manager to adjust their visibility preferences by clicking the "eye" icon in the Options Toolbar and selecting or deselecting columns. Once set, visibility preferences will be persisted. Any future query containing columns that were previously shown or hidden by the user will also reflect these changes. The user can access the Column Visibility Manager to make changes to these visibility preferences at any time.
enableQueryInterpretation
: Query interpretation is a reverse translation of how AutoQL interpreted the query being asked returned to the user. This feature can be helpful in determining whether or not a query is being understood the way that it was meant to.
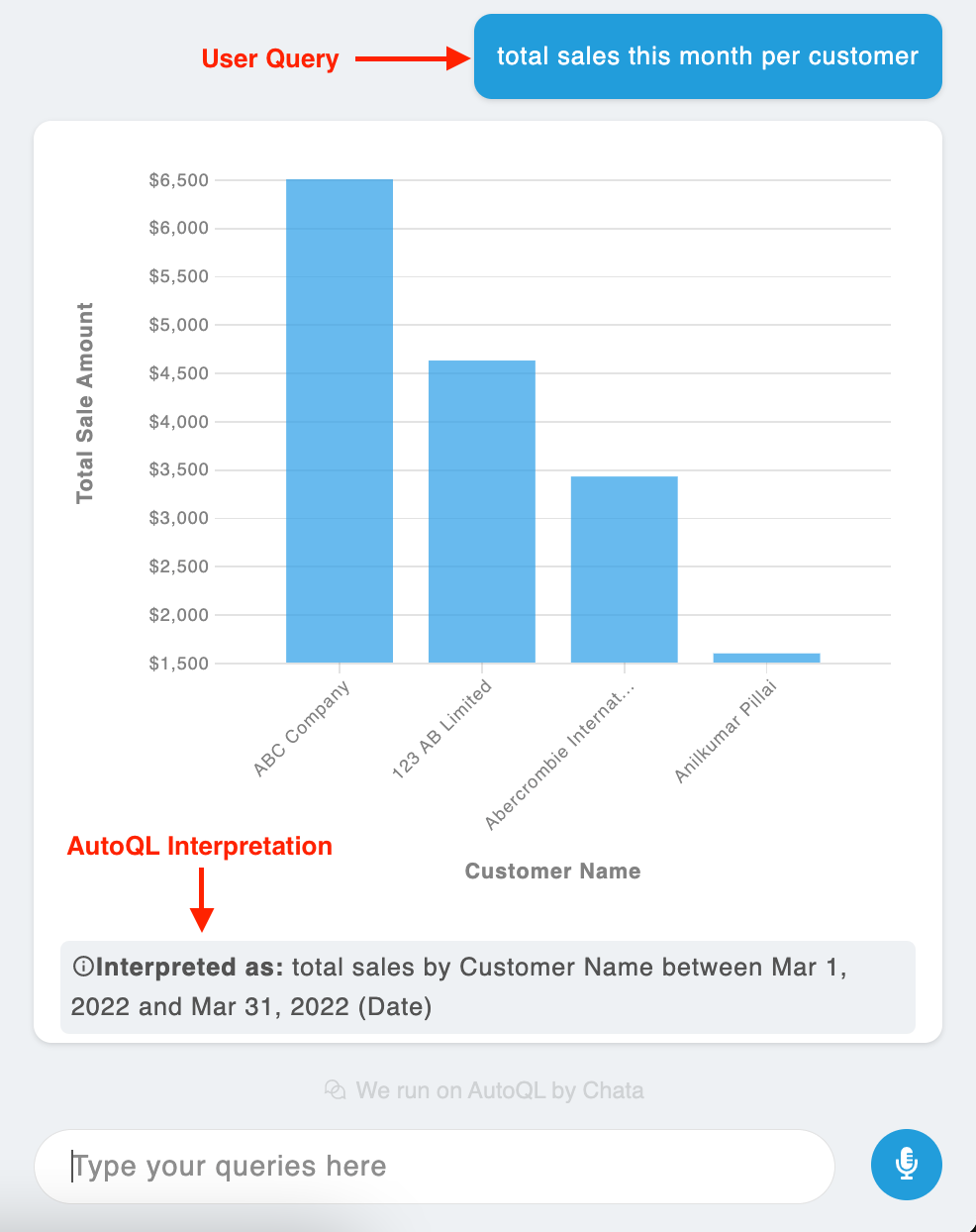
defaultShowInterpretation
: This will determine whether or not the Query Interpretation box is expanded or collapsed by default. Note: This is only applied when enableQueryInterpretation
is set to true.
enableFilterLocking
: Filter Locking allows users to set a context for your queries to be situated around based on value labels that exist within the database. If set to true, a lock icon will become visible in the header bar that opens a GUI for managing these filters. From here they can be searched via the input bar, and once selected, can be stored per user (Persistent) or for the duration that the browser tab is open (Session).
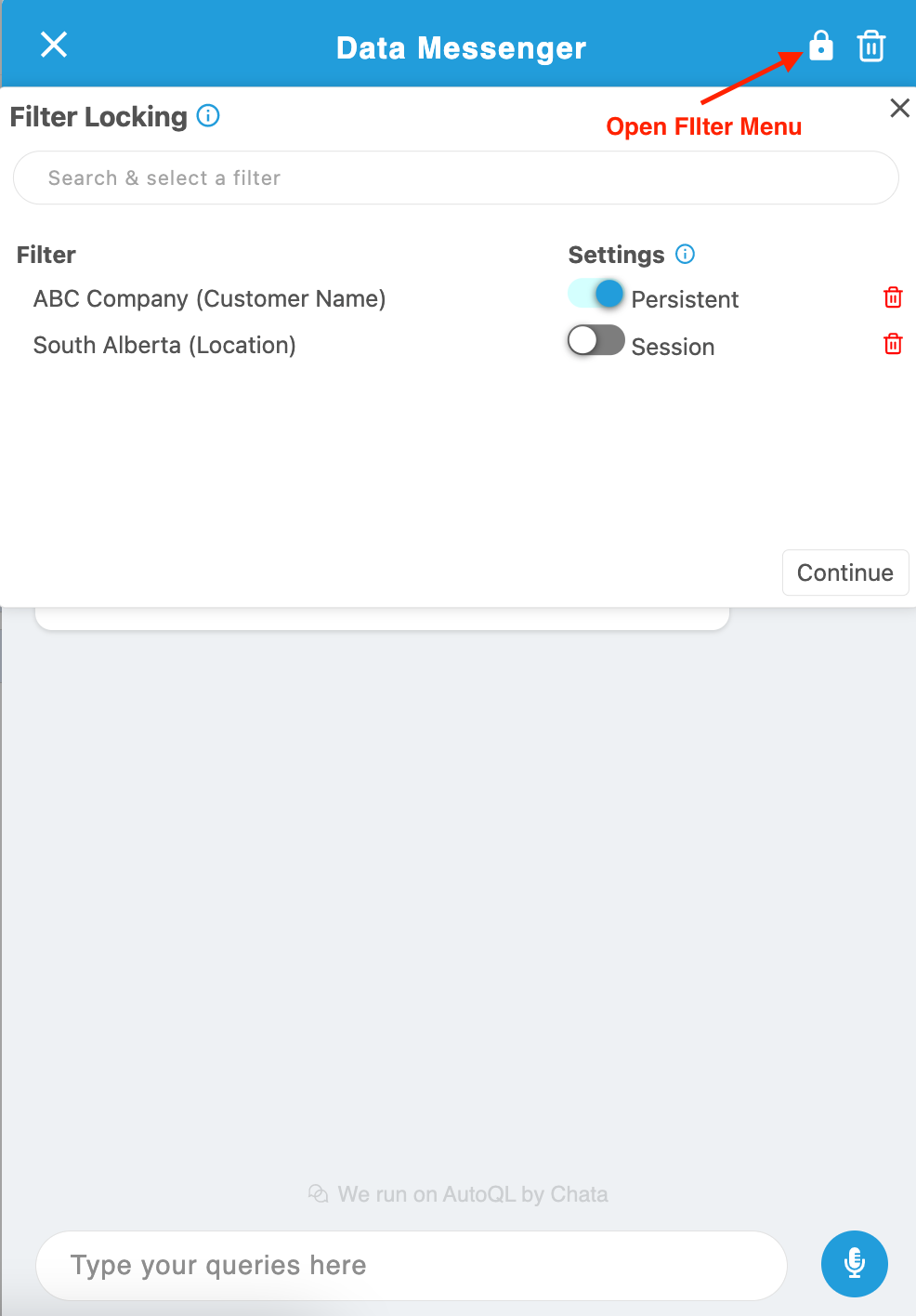
enableNotifications
: Enabling this allows notifications to be polled if enableNotificationsTab
is also enabled.
enableCSVDownload
: This opens up the "Download as CSV" option in the "More options" menu that becomes visible when hovering over a query response.
debug
: If this value is true, the user can copy the full query language (QL) statement (ex. SQL statement) that was dynamically generated from their natural language query input by clicking "Copy generated query to clipboard".
dataFormatting Prop
Key | Value Type | Default |
---|---|---|
currencyCode | String | 'USD' |
languageCode | String | 'en-US' |
currencyDecimals | Number | 2 |
quantityDecimals | Number | 1 |
comparisonDisplay | String: 'PERCENT' || 'RATIO' | 'PERCENT' |
monthYearFormat | String | 'MMM YYYY' |
dayMonthYearFormat | String | 'll' |
currencyCode
: If your data is not in USD, you can specify a different currency code here. All visualizations (tables and charts) will show the default currency formatting for the specified code.
Currency
Setting a currency code does not perform a currency conversion. It only displays the number in the desired format.
languageCode
: If the currency code from your country requires letters not contained in the English alphabet to show symbols correctly, you can pass in a locale here. For more details on how to do this, visit: https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/NumberFormat
currencyDecimals
: Number of digits to display after a decimal point for currencies.
quantityDecimals
: Number of digits to display after a decimal point for quantity values.
comparisonDisplay
: Format for displaying comparison types (ex. changes in data). "Percent" will multiply the number by 100 and display a "%" symbol. "Ratio" will display the original number as-is.
monthYearFormat
: The format to display the representation of a whole month. (ex. March 2020). AutoQL uses dayjs for date formatting, however most Moment.js formatting will work here as well. Please see the dayjs docs for formatting options.
dayMonthYearFormat
: The format to display the representation of a single day. (ie. March 18, 2020). AutoQL uses dayjs for date formatting, however most Moment.js formatting will work here as well. Please see the dayjs docs for formatting options.
themeConfig Prop
Key | Value Type | Default |
---|---|---|
theme | String: 'light' || 'dark' | 'light' |
accentColor | String | light theme: '#28a8e0', dark theme: '#525252' |
accentTextColor | String | light theme: '#ffffff', dark theme: '#ffffff' |
fontFamily | String | 'sans-serif' |
chartColors | Array | ['#26A7E9', '#A5CD39', '#DD6A6A', '#FFA700', '#00C1B2'] |
theme
: Color theme for Data Messenger. Currently there are two options: light theme and dark theme. In addition to changing the overall theme, you can also change the accent color using the accentColor prop.
accentColor
: Primary accent color used in Data Messenger. This is the color of the messages displayed in the interface (both natural language query inputs from users and the associated responses that are generated and returned to the user). The visualization (table and chart) colors will not be affected here.
accentTextColor
: Primary accentTextcolor used in Data Messenger. This is the color of DataMessenger icon, the header, speech-to-text button, and the some other buttons' icon inside of DataMessenger. The visualization (table and chart) colors will not be affected here. If this prop is not supplied, then the accentTextcolor will be black or white, determined by the brightness of accentColor. For example, if the accentColor are dark, then the accentTextColor will be white, otherwise black.
fontFamily
: Customize the font family to the provided font wherever possible. Accepts any CSS font family that is available, and if none is provided, will default to Sans-Serif.
chartColors
: Array of color options for the chart visualization themes, starting with the most primary. You can pass any valid CSS color format in here, however it is recommended that the color is opaque (ex. "#26A7E9", "rgb(111, 227, 142)", or "red"). Charts will always apply the colors in order from first to last. If the visualization requires more colors than provided, all colors will be used and then repeated in order.
Examples
import React, { Component } from 'react'
import { DataMessenger } from 'react-autoql'
import 'react-autoql/dist/autoql.esm.css'
export default class App extends Component {
render = () => {
return (
<DataMessenger
authentication={{
apiKey: "your-api-key"
domain: "https://yourdomain.com"
token: "yourToken"
}}
placement="bottom"
maskClosable
showMask={false}
width="700px"
handleStyles={{ left: 'unset', right: '25px' }}
/>
)
}
}
Updated about 2 years ago